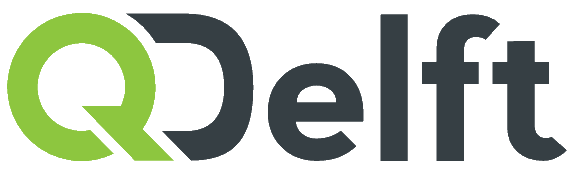
React Accessibility
Tooling and techniques
- Almero Steyn
- QDelft B.V.
- almerosteyn.com
- twitter.com/kryptos_rsa
"A JavaScript library for building user interfaces." - React docs
import React from 'react';
import Content from './Content';
const Root = ({headerText, onLogout}) => (
{ headerText }
<Content onLogout={ onLogout }/>
);
export default Root;
yarn global add create-react-app
create-react-app my-react-app
cd my-react-app
yarn start
HTML-like syntactic JavaScript sugar.
<label htmlFor={nameId}>{nameLabelText}</label>
<input id={nameId} type="text" />
Renders to HTML in the DOM.
All ARIA attributes are valid JSX props!
<input id={nameId} aria-label={accessibleLabel} type="text" />
IntelliSense in supported IDEs.
"Static AST checker for a11y rules on JSX elements."https://github.com/evcohen/eslint-plugin-jsx-a11y
More than 30 ESLINT a11y checks.
Rules are configurable.
{
"rules": {
"jsx-a11y/rule-name": "warn"
}
}
Extending config in create-react-app.
{
"extends": ["react-app", "plugin:jsx-a11y/recommended"],
"plugins": ["jsx-a11y"]
}
A11y issues become build warnings.
IDE integration.
"Accessibility auditing for React.js applications."
Setting up react-axe.
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
if (process.env.NODE_ENV !== 'production') {
const axe = require('react-axe');
axe(React, ReactDOM, 1000);
}
ReactDOM.render(<App />, document.getElementById('root'));
Browser console feedback.
Setting focus with refs.
componentDidMount(){
this.nameInput.focus();
}
render(){
return(
<input id="demoId" type="text"
ref={(input) => {this.nameInput = input;}}/>
);
}
class App extends Component {
state = { LazyBlock: null };
async componentDidMount() {
const { default: LazyBlock } = await import('./LazyBlock');
this.setState({ LazyBlock: <LazyBlock/> });
}
render() {
return ( <main aria-busy={!this.state.LazyBlock}>
{this.state.LazyBlock || Loading...
}
</main> );
}
}
Splitting code files.
Presentation online at:
http://almerosteyn.com/slides/