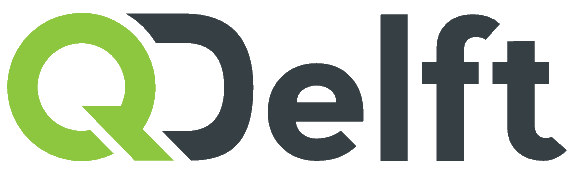
React Accessibility Patterns
- Almero Steyn
- QDelft B.V.
- almerosteyn.com
- twitter.com/kryptos_rsa
"A JavaScript library for building user interfaces." - React docs
<div className="looks-like-a-button"
onClick={this.onClickHandler}>
Press me please
</div>
import React, { Component } from 'react';
class Demo extends Component {
render() {
return (
{this.props.displayText}
);
}
}
export default Demo;
import React from 'react';
const Demo = ({ displayText }) => (
{displayText}
);
export default Demo;
import React from 'react';
import Demo from './Demo';
const Root = () => (
<Demo displayText="Show this text"/>
);
export default Root;
HTML-like syntactic JavaScript sugar.
<label htmlFor={nameId}>{nameLabelText}</label>
<input id={nameId} type="text" />
Renders to HTML in the DOM.
const AppNavigation = () =>
;
Bad idea:
<div className="looks-like-button"
onClick={this.onClickHandler}>
Press me
</div>
Good idea:
<button onClick={this.onClickHandler}>
Press
</button>
const HeaderWithLevel = ({ headerText, level }) => {
const HeaderLevel = `h${level}`;
return <HeaderLevel>{headerText}</HeaderLevel>;
};
import uuid from 'uuid';
//...
this.inputId = uuid.v4();
//...
render() {
//...
<label htmlFor={this.inputId}>
{labelText}
</label>
<input id={this.inputId}
onChange={this.onChangeHandler}
value={value}
/>
//...
}
https://github.com/kelektiv/node-uuid
const AppMain = () =>
<Switch>
<Route path="/todos" component={Todos} />
<Route path="/todo" component={Todo} />
<Route path="/contact" component={Contact} />
<Redirect to="/todos" />
</Switch>
;
class PageFocusSection extends Component {
componentDidMount() {
this.header.focus();
}
render() {
const { children, headingText } = this.props;
return (<section>
<h2 tabIndex="-1" ref={header => (this.header = header)}>
{headingText}
</h2>
{children}
</section>);
}
}
//...
import DocumentTitle from 'react-document-title';
//...
const SetDocTitle = ({ docTitle, children }) =>
<DocumentTitle title={docTitle}>
{children}
</DocumentTitle>;
https://github.com/gaearon/react-document-title
//...
import { LiveAnnouncer, LiveMessage } from 'react-aria-live';
//...
render() {
return (
<LiveAnnouncer>
<LiveMessage message={this.state.a11yMessage}
aria-live="polite" />
{this.props.children}
</LiveAnnouncer>
);
}
//..
https://github.com/AlmeroSteyn/react-aria-live
Presentation online at:
http://almerosteyn.com/slides/